Security Access#
The automated system state reverse-engineering feature of HydraScope can automatically detect security-access levels of an ECU. If a security access algorithm is provided to HydraScope, new system states can automatically be detected and UDS scans can be performed in those.
Since the security access algorithm is crucial for the overall security of an ECU, we provide a simple and secure way to extend HydraScope with a custom security access algorithm.
Overview#
To extend HydraScope with a custom security access algorithm, a small server application which provides the security access key for a given seed that can be implemented quickly on a user’s computer.
During a UDS scan, HydraScope tries to retrieve seeds for all possible security access levels of an ECU under test. Once HydraScope detects a seed, this seed is forwarded to the custom security access server as an HTTP post containing the following JSON data blob.
{'seed': '5eed01020304', 'access_level': 1}
This HTTP post expects the security access key as answer inside a JSON data blob.
{'security_access_key': '7337'}
Custom Security Access Server#
A simple security access server can be implemented in python as seen in the example below.
from flask import Flask, json, request
api = Flask(__name__)
@api.route('/security_access',methods = ['POST'])
def security_access():
try:
json_request = request.json
seed = json_request['seed']
seed_bytes = bytes.fromhex(seed)
access_level = json_request['access_level']
if access_level == 1:
key = bytes(reversed(seed_bytes))
else:
key = b"\xff\xff\xff\xff"
return {'security_access_key': bytes.hex(key)}
except Exception as e:
print(e)
return {}
if __name__ == '__main__':
api.run()
To start this server on you local machine, enter the following line in the terminal, assuming you saved the above
code in a file called security_access.py
python security_access.py
In HydraScope, just enter the URL of your server in the UDS-Scan settings.
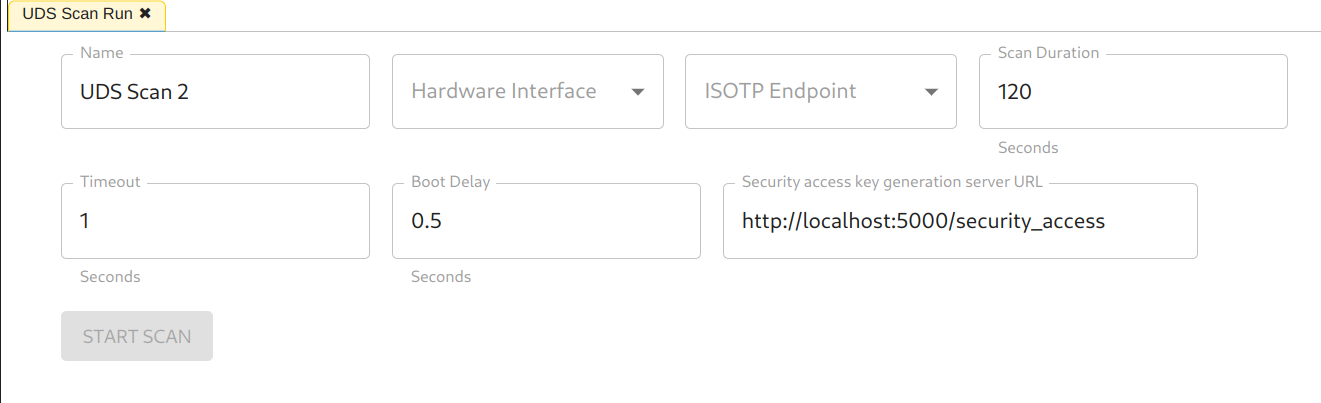
Fig. 1 UDS Scan configuration with custom security access server.#