Message Authentication (AUTOSAR SecOC)#
HydraVision - Automated Testing with HydraVision from dissecto.
Penetration Testing - Consulting Are you looking for a pentest?? Please have a look at our service offerings.
AutoSAR (Automotive Open System Architecture) SecOC (Security On-board Communication) is a security architecture that aims to protect the communication between the various electronic control units (ECUs) within a vehicle against cyber-attacks.
SecOC is an AUTOSAR module
Provides integrity and authentication for messages (PDUs)
Freshness protects against replay attacks
Generic specification which can operate with asymmetric or symmetric cryptography
Key distribution is not specified
Every PDU has a unique identifier (SecOCDataID).
On CAN networks, the CAN identifier is used.
On Ethernet networks, the PDU identifier or internal mappings for PDU identifier to SecOCDataIDs are used.
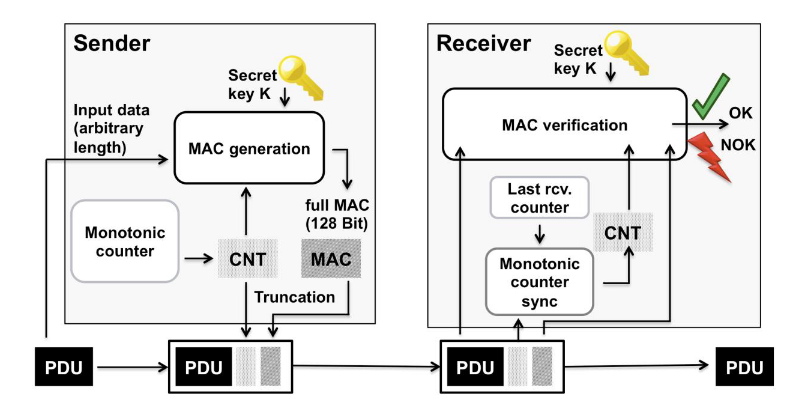
Fig. 37 Overview SecOC. Author: AUTOSAR#
Figure from [AUT20].
Generation#
A Secured I-PDU contains freshness value and the MAC
Freshness value is incremented on every transmit or derived from a tick count
MAC generation input is the SecOCDataID, the PDU, and the freshness value.
In symmetric mode, MAC bits can be chopped off. The security level decreases linearly with the MAC size.
Truncation#
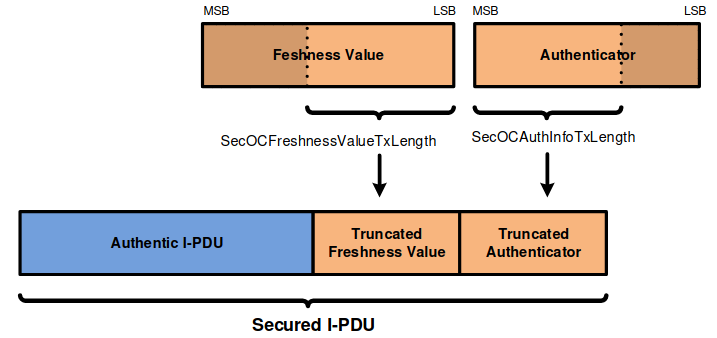
Fig. 38 Secured I-PDU contents with truncated Freshness Counter and truncated Authenticator. Author: AUTOSAR#
Figure from [AUT20].
MAC and freshness value are only transferred in truncated format.
Truncation saves bandwidth
Verification#
Only LSBs of the freshness value are transmitted
Compute full freshness value for internal purposes.
Overwrite LSBs of last received value
If received LSBs smaller than the last LSBs, increment MSBs
Calculate MAC from PDU, full freshness count
If calculated and transmitted MACs match, accept PDU otherwise reject.
Profiles#
AutoSAR specifies three different profiles with different sizes for truncated freshness value and truncated MAC. All profiles use CMAC with AES128
SecOC Profile 1 (or 24Bit-CMAC-8Bit-FV)
Algorithm: CMAC/AES-128
truncated freshness value: 8 bits
truncated MAC: 24 bits
SecOC Profile 2 (or 24Bit-CMAC-No-FV)
Algorithm: CMAC/AES-128
truncated freshness value: 0 bits
truncated MAC: 24 bits
Don’t use freshness values at all
SecOC Profile 3 (or JASPAR)
Algorithm: CMAC/AES-128
length of freshness value: 64 bits
truncated freshness value: 4 bits
truncated MAC: 28 bits
Freshness Value#
The freshness value is necessary to protect against reply attacks. The AUTOSAR standard recommends the following structure of the freshness value. The ‘Reset Flag’ field is represented by the two lowest bits of the ‘Reset Counter’ and incremented on every send of a Sync Message, respectively on every increment of the ‘Reset Counter’.
Next to the standards’ recommendation, a common practice is to distribute a monotonic tick count across all ECUs via authenticated PDUs.
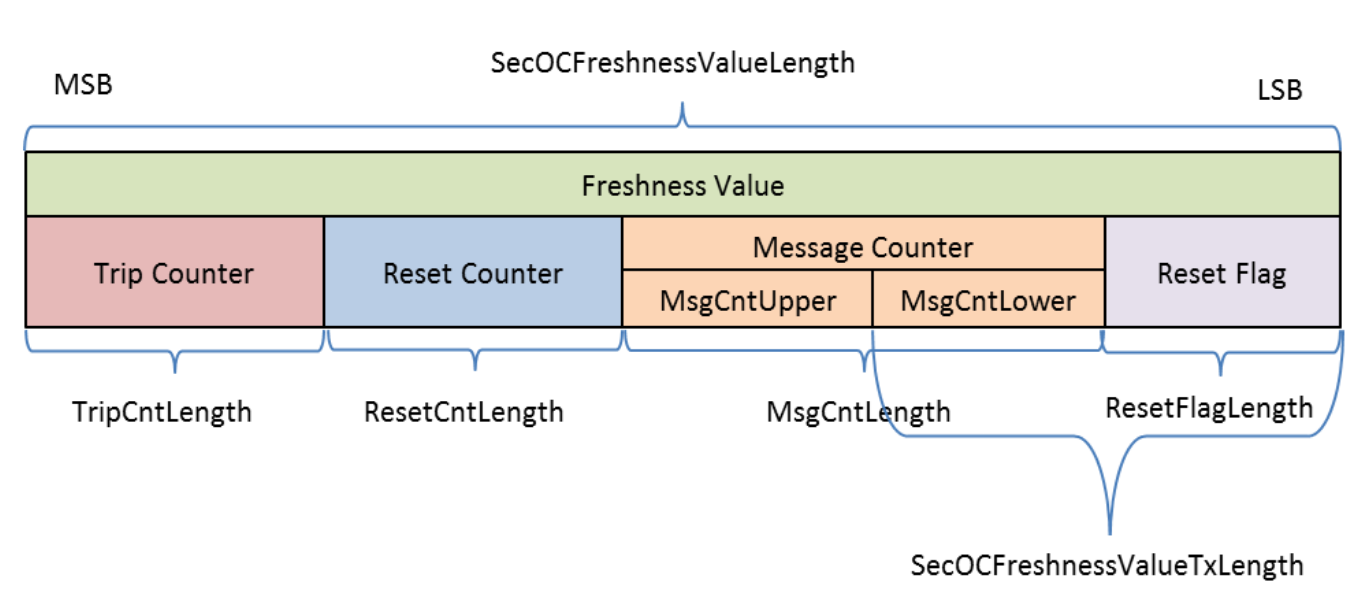
Fig. 39 Structure of FreshnessValue. Author: AUTOSAR#
Figure from [AUT20].
Sync Message#
Synchronization messages will distribute the ‘Trip Counter’ and the ‘Reset Counter’ across all ECUs in the vehicle. This is necessary to preserve an identical freshness value across all ECUs.
According to the standard, a Sync message is sent, every time the ‘Message Counter’ overflows.
Security Recommendation:
To ensure the proper distribution of Sync Messages, it’s crucial that ‘Sync Messages’ can only be sent via Broadcast or Multicast. If an attacker can send authenticated Unicast ‘Sync Messages’, a Denial of Service (DoS) Attack against the AUTOSAR SecOC communication of one single ECU can be launched. Suppose, the ‘Sync Message’ is protected via a CMAC, similar to SecOC PDUs, this would result in the secret key of the ‘Sync Message Authenticator’ being shared across all ECUs of a vehicle, resulting in the fact, that the weakest ECU can be used by an attacker to leak the secret key [Mel24].
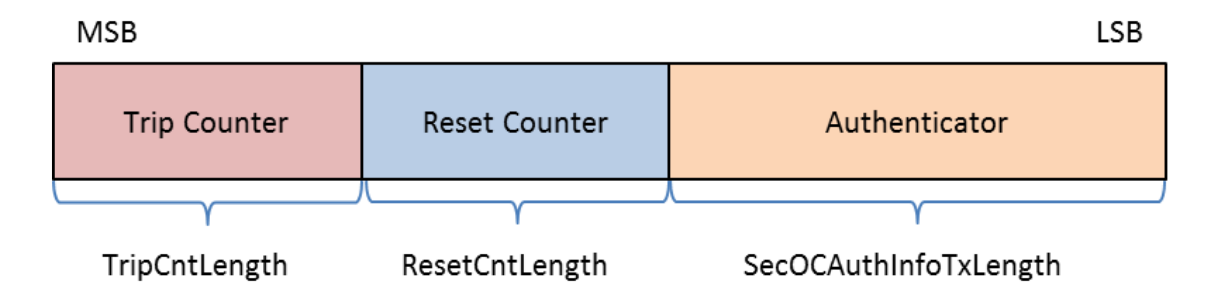
Fig. 40 Format of the synchronization message (TripResetSyncMsg). Author: AUTOSAR#
Figure from [AUT20].
Key Management#
Some potential solutions:
One global key
ECUs can be swapped easily.
If keys are leaked, attackers can attack all systems
One key per vehicle
Keys need to be loaded on an ECU, if components are swapped.
If the attacker gains code execution, any message can be sent.
Protects bus from third-party devices
One key per message
Ideal if the asymmetric mode is used, but usually not practical
In symmetric mode: A hacked ECU can send malicious frames of all messages it receives and sends in normal operation.
Requires a lot of keys
Takeaways:
SecOC does not protect against RCE attacks in most cases, only ensures the authenticity of PDUs.
Shared secrets depend on the security of the weakest link (ECU).
Side-Channel-Attacks against software implementations of AES are practical. Attackers can trigger and modify most of the input of the CMAC algorithm.
SecOC in Scapy#
Scapy supports dissection, build, verification and authentication of SecOC messages, sent via AUTOSAR PDUs or CANFD packets.
General difficulties for the implementation#
As so often, every OEM defines their own standard how SecOC is implemented. Therefore, the implementation in Scapy needs to be vendor independent and easy customizable.
The freshness value needs to be tracked
The SecOCDataID needs to be known
SecOC PDUs are “mixed” with non-SecOC PDUs, only differ by identifier.
Customization#
To address the common difficulties, Scapy SecOC Packets have three stub functions that need to be overwritten in order to use SecOC properly. The following code example explains the customization capabilities.
# The following code is pseudocode and only for explanatory purposes.
class My_SecOC_CANFD(SecOC_CANFD):
def get_secoc_payload(self) -> bytes:
"""
This method is used by 'get_message_authentication_code'
to get the payload including SecOCDataID for MAC computation.
"""
secoc_data_id = self.identifier # CANFD identifier
payload = self.pdu_payload
raise bytes(secoc_data_id) + bytes(payload)
def get_secoc_key(self) -> bytes:
"""
This method can be used to provide an individual secret key for every secoc_data_id
"""
secoc_data_id = self.identifier
secoc_key = GLOBAL_KEYS[secoc_data_id]
return secoc_key
def get_secoc_freshness_value(self) -> bytes:
"""
This method is used to provide the full freshness value for MAC computation
"""
freshness_value = trip_count + reset_counter + message_count + self.tfv
return bytes(freshness_value)
Preparation#
In order to use Scapy to dissect SecOC and non-SecOC AUTOSAR PDUs or CANFD frames properly, SecOC PDUs need to be registered so that the dissector knows, weather to use the SecOC variants or the non-SecOC variants of the Packet for dissection. The following code example will explain the necessary code.
My_SecOC_CANFD.register_secoc_protected_pdu(pdu_id=0x123)
socket = CANSocket("vcan0", fd=True, basecls=My_SecOC_CANFD)
This line will tell the dissector to dissect any incoming packet with identifier == 0x123 as SecOC_CANFD packet. All other packets will be interpreted as CANFD packets.
Working with SecOC#
Once we obtain a SecOC Packet from a socket or a PCAP file, we can use the SecOC related functions of the Packet. The following possibilities are given:
# suppose this is our SecOC packet
pkt: My_SecOC_CANFD
# a call to secoc authenticate will update the truncated freshness value and the truncated message authentication code of the packet
pkt.secoc_authenticate()
print(pkt.tfv) # is now updated
print(pkt.tmac) # is updated as well
# a call to secoc verify will compute the message authentication code from the payload of the packet and the local freshness value and will compare the truncated message authentication code of the packet.
if pkt.secoc_verify() == True:
print("Message verified")
Real-World Issues in SecOC Implementations#
SecOC implementations encounter various practical issues that compromise both security and functionality:
Inconsistent MAC Generation: Confusion around which fields are included in the Message Authentication Code (MAC)—such as CAN-ID, PDU ID, or the length field—leads to unpredictable security behavior.
Custom Freshness and Sync Mechanisms: Custom Freshness Values (FV), sync protocols, and handling of FV rollovers introduce inconsistencies across implementations.
Key Management Complexities: Variability in key derivation, storage, updates, and PDU-ID to key mapping affects secure key handling practices, compromising communication integrity.
These challenges highlight the need for standardized SecOC practices to improve security and reliability in automotive communications.